GitHub Workflow: Managing CI/CD for Multi-Organisation Forked Repositories
Efficiently manage CI/CD workflows for forked GitHub repositories across multiple organizations with branching strategies and precise workflow triggers.
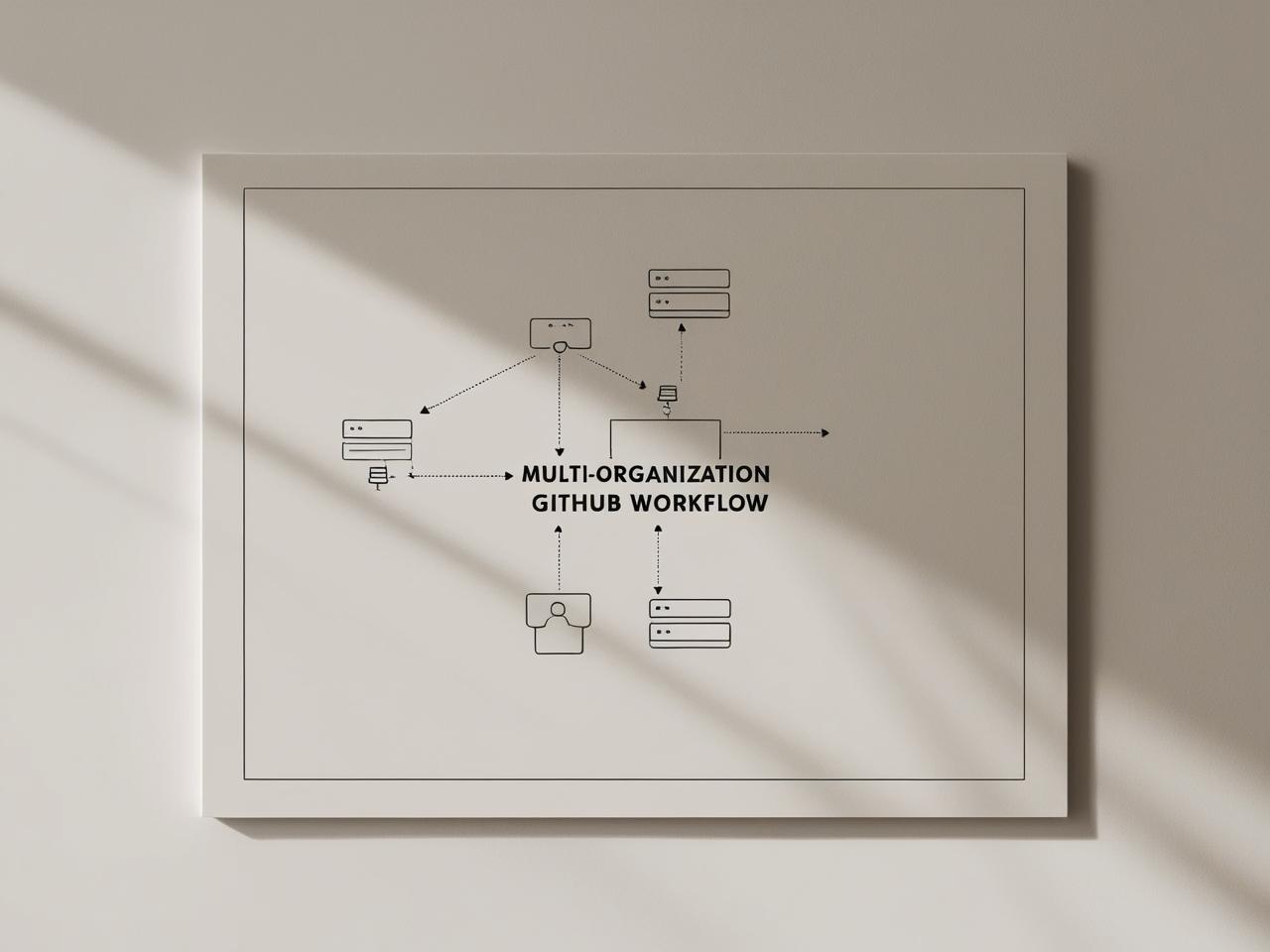
The Challenge of Multi-Organization Workflows
Managing CI/CD workflows across forked repositories presents unique challenges. When Organization A maintains the upstream repository and Organization B maintains a fork, we need to ensure that:
- Workflows run in the correct repository context
- Resources aren’t wasted by duplicate workflow runs
- Each organization maintains control over its CI/CD processes
- Pull requests and merges work smoothly across organizations
Let’s explore how to achieve this through proper configuration and strategic workflow design.
Implementing Organization-Specific Workflows
Base Workflow Structure
First, let’s look at a basic workflow that runs only in the upstream repository:
# .github/workflows/upstream-ci.yml
name: Upstream CI
on:
push:
branches: [main]
pull_request:
branches: [main]
jobs:
build:
if: github.repository == 'orgA/upstream-repo'
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Set up environment
run: |
echo "Running in upstream repository"
echo "REPO_TYPE=upstream" >> $GITHUB_ENV
- name: Run upstream-specific tests
run: |
if [[ "$" == "pull_request" ]]; then
echo "Running PR validation suite"
else
echo "Running full test suite"
fi
Fork-Specific Workflow
For the forked repository, we’ll create a separate workflow with organization-specific checks:
# .github/workflows/fork-ci.yml
name: Fork CI
on:
push:
branches: [main, feature/*]
pull_request:
branches: [main]
jobs:
build:
if: github.repository_owner == 'orgB'
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
- name: Configure fork environment
run: |
echo "Running in forked repository"
echo "REPO_TYPE=fork" >> $GITHUB_ENV
- name: Run fork-specific validations
run: |
if [[ "$" =~ ^refs/heads/feature/ ]]; then
echo "Running feature branch validation"
else
echo "Running standard validation"
fi
Advanced Workflow Orchestration
Composite Action for Shared Logic
Create reusable workflow components using composite actions:
# .github/actions/shared-validation/action.yml
name: 'Shared Validation Steps'
description: 'Common validation steps for both upstream and fork'
inputs:
repo-type:
description: 'Type of repository (upstream/fork)'
required: true
runs:
using: "composite"
steps:
- name: Run common validation
shell: bash
run: |
echo "Running shared validation for $"
- name: Security scan
shell: bash
run: |
echo "Running security checks"
- name: Code quality
shell: bash
run: |
echo "Running linting and style checks"
Pull Request Workflow
Here’s a specialized workflow for handling pull requests between organizations:
# .github/workflows/cross-org-pr.yml
name: Cross-Organization PR
on:
pull_request:
types: [opened, synchronize, reopened]
branches: [main]
jobs:
validate:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
with:
fetch-depth: 0
- name: Validate PR source
id: pr-check
run: |
if [[ "$" != \
"$" ]]; then
echo "Cross-organization PR detected"
echo "is_cross_org=true" >> $GITHUB_OUTPUT
else
echo "Internal PR detected"
echo "is_cross_org=false" >> $GITHUB_OUTPUT
fi
- name: Run cross-org validation
if: steps.pr-check.outputs.is_cross_org == 'true'
run: |
echo "Running additional validation for cross-org PR"
# Add specific validation steps here
Branching Strategy
Implement a clear branching strategy that works across organizations:
- Main Branch Protection
# Branch protection rules in GitHub repository settings branches: main: protect: true required_status_checks: strict: true contexts: ["Upstream CI", "Fork CI"] required_pull_request_reviews: required_approving_review_count: 2
- Feature Branch Convention
- Format:
feature/<org>-<description>
- Example:
feature/orgB-user-authentication
- Format:
- Synchronization Branch
- Dedicated
sync-upstream
branch in forks - Regular automated sync using GitHub Actions:
- Dedicated
# .github/workflows/sync-upstream.yml
name: Sync with Upstream
on:
schedule:
- cron: '0 0 * * *' # Daily at midnight
workflow_dispatch:
jobs:
sync:
if: github.repository != 'orgA/upstream-repo'
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v3
with:
fetch-depth: 0
- name: Configure Git
run: |
git config user.name github-actions
git config user.email github-actions@github.com
- name: Sync with upstream
run: |
git remote add upstream https://github.com/orgA/upstream-repo.git
git fetch upstream
git checkout main
git merge upstream/main
git push origin main
Best Practices and Optimization
1. Resource Management
- Use conditional job execution to avoid redundant runs
- Implement caching for dependencies and build artifacts
- Set appropriate timeout limits for workflows
2. Security Considerations
- Use environment secrets for organization-specific credentials
- Implement CODEOWNERS for critical paths
- Regular security scanning in both upstream and fork
3. Workflow Optimization
- Use matrix builds for parallel testing
- Implement intelligent test splitting
- Cache dependencies and build artifacts
Common Pitfalls and Solutions
- Duplicate Workflow Runs
- Solution: Use conditional checks based on repository context
- Implement proper event filtering
- Permission Issues
- Solution: Use GitHub Apps for authentication
- Implement fine-grained access controls
- Synchronization Conflicts
- Solution: Automated conflict detection
- Clear resolution procedures
Conclusion
A well-designed multi-organization GitHub workflow requires careful consideration of repository contexts, proper event handling, and clear branching strategies. By implementing the patterns and practices outlined above, teams can achieve efficient collaboration while maintaining security and control over their CI/CD processes.
References
- GitHub Actions Documentation
- GitHub Flow Guide
- GitHub Actions: Creating Composite Actions
- Branch Protection Rules
sitemap:
priority: 0.8
lastmod: 2024-08-10
changefreq: monthly